![]() |
Welcome to the AstrOmatic development blog. Here we talk software, hardware, systems, web technology and other nerdy stuff related to AstrOmatic. |
Solving C library call incompatibilities with the dynamic linking loader
While testing the latest dynamically-linked binary version of SCAMP on various Linux platforms, I discovered that the executable would sometimes exit with the error message:
This happens exclusively with older versions of the PLplot library, libplplotd, because of a change PLplot developers made to the API circa 2007. After this date, plimage() was replaced with c_plimage(). Although compatibility is maintained at compile time thanks to a
in the plplot.h include file, it is lost for dynamically-linked executables such as SCAMP. How then to make the program work with any version of the PLplot library? To my knowledge, there isn’t much that can be done at compile or link-time using the standard C library to solve this problem.
Fortunately, a POSIX-compliant solution exists. The dlopen(), dlclose(), and dlsym() functions allow respectively to open/close a dynamic library, and query symbol (function) pointers in it. The problem above can be solved by replacing calls to plimage() by (*myplimage)(), and setting the function pointer myplimage either to plimage or c_plimage depending on their availability. Here is a code example to replace a call to plimage(3.1416) (I simplified the arguments to plimage() for readability):
…
void (*myplimage)(double *dummy);
void *dl, *fptr;
dl = dlopen("libplplotd.so", RTLD_LAZY);
if ((fptr = dlsym(dl, "plimage")))
myplimage = fptr;
else
myplimage = dlsym(dl, "c_plimage");
(*myplimage)(3.1416);
dlclose(dl);
…
RTLD_LAZY is one of the flags available to control binding rules; see the dlopen() man page for more details.
Using the INTEL C compiler
As already reported here and here, scientific code compiled with the Intel C compiler (icc) used to get a “free” 10-30% speed boost compared to code compiled with the Gnu compiler (gcc), thanks mostly to Intel’s vectorised math routines. gcc is now at version 4.4, and icc at version 11.1, and… this remains true, in both 32 and 64 bits. Therefore most AstrOmatic RPM packages are still compiled with icc. Two things should be mentioned:
- Using the latest version of the Intel C compiler has one drawback: older x86 processors (older than Pentium III) are not supported.
- The icc version used for compiling AstrOmatic packages has been patched to deactivate Intel CPU checks. As already shown here and later confirmed by Intel, the Intel math library happens to deactivate streaming SIMD instructions on non-Intel CPUs, which can result in a 10+% speed drop on AMD processors. The Swallowtail web site used to have a page on the subject, and to propose patches, but they do not seem available anymore. I hopefully kept copies which you can find for download below. These are Perl scripts written by M.D. Mackey. They seem to work for all icc versions from 7.x to 11.x. The first one is for executables compiled with icc, while the second one will patch the compiler libraries once for all.
Optimising web page access speed
Thanks to high-speed internet networks and efficient caching techniques, web pages can now be transmitted around the world in a fraction of a second. However, the spread of Web 2.0 technology over the past years has generalised the extensive use of large CSS sheets and AJAX code, and web pages that rely on several hundreds of kbytes of Javascript code are not uncommon these days. If no care is taken, risks that a web site with rich, dynamic content generates waiting times of many seconds for every page accessed are high, even from a high-speed connection. Despite all its bells and whistles, browsing such a web site may lead to a rather frustrating experience.
Hopefully, ways to optimise web-page access efficiency exist. A good start is to use a network performance measurement tool. There are several plug-ins for web browsers around. On Firefox, the combination of Firebug and Yslow will give you a wealth of statistics each time you access a web-page, and provide hints about what parts of the served content require most attention. Putting aside optimising server-side generation of dynamic content and cache management, one way to significantly reduce access time is to compress web-page content, especially CSS sheets and Javascript programs. Both of them are ASCII files, and may greatly benefit from compression. Compression can be achieved through
- “minification“, which consists of making the code more compact by stripping unnecessary characters and reducing the length of local symbols. Popular offline minification tools include JSMin and Yahoo’s YUI Compressor. Both tools do a great job at reducing the file sizes (gains of 30% are not uncommon). The processed files are almost unreadable, but hopefully perform otherwise exactly the same. To avoid maintaining both uncompressed and compressed versions of the same code, on-the-fly minification tools have been written in PHP: see for instance JSMin-php or Minify!.
- generic file-compression. The possibility to send compressed content has been part of the HTTP protocol since 1999 (HTTP 1.1). Web servers can compress content on-the-fly before sending it to browsers that support it. For content consisting mostly of ASCII text ((X)HTML, CSS or JavaScript), reduction in file size, and therefore bandwith increase can be large: a compression ratio of 5× is pretty typical. Compression and decompression put more stress on servers and clients; but compression is generally beneficial with modern processors, at least for websites under moderate load. Problems with compressed content seem to occur for some older browsers and proxies. Fortunately, compression server modules such as Apache2‘s mod_deflate can be configured precisely to compress specific file types for specific clients.
Both compression techniques applied to the AstrOmatic website, reduce the total number of bytes sent for AstrOmatic pages (before caching!) from an average 800kB to a more reasonable 400kB (including 310kB of image data 😆 ).
Displaying math in web pages
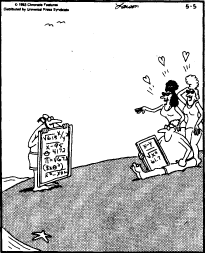
For many years, displaying good-looking mathematical formulas in a web page would require little short of a small rendering pipeline which would include for instance the LaTeX typesetting system and a rasteriser such as Ghostscript or dvipng. Then came the MathML language, an application of XML for describing mathematical expressions. The Gecko layout engine brought built-in MathML support to gecko-based web browsers (including Netscape 7-9, Mozilla and Firefox), for the first time in 2002. However MathML content does not look always good by default (especially square roots); one needs to install the STIX fonts first, which for some reason are not part of the Firefox package yet.
As of March 2009 few other browsers offer proper native MathML support. Microsoft Internet Explorer (at least up to version 8) requires the MathPlayer plugin to be able to display MathML. The Opera browser supports a restricted part of (presentational) MathML3 through CSS formatting since release 9.5 in 2008. The WebKit engine that powers Apple Safari does not have native MathML support, but some people have managed to display MathML in Safari and even on the iphone using the appropriate XSLT filter and css stylesheet (MathML profile for css). You may check the level of MathML support in your browser by visiting this page or this one.
Another problem is that MathML, like other XML-based languages, is not very human-readable; and typing a complex equation directly in MathML is not an enjoyable experience. Thus anyhow one must still rely on LaTeX and its clear, standardised syntax for entering equations in blogs or copying them straight from scientific paper LaTeX sources. There are several LaTeX to MathML translators out there, particularly itex2mml and TeX4ht, which can be used on the server side. The alternative road is to do the transcription job on the client side, using JavaScript. ASCIIMathML is a clever JavaScript code which makes tour browser automatically detect LaTeX-like expressions in the current web page and translate them into MathML. The nice thing with it is that one does not have to care about DOCTYPEs and Namespaces in the XHTML declaration; it can even be used with plain HTML documents. The main disadvantage is that it adds about 100kB of code to your webpage content (which will hopefully be cached the next time you access similar pages).
The AstrOmatic website uses a modified version of ASCIIMathML that offers fallback to generating PNG images for browsers that do not support MathML. Here is below the expression of polar shapelet basis vectors which should display nicely on all recent browsers:
$\psi_{n,m}(r,\theta) = \frac{(-1)^{\frac{n-|m|}{2}}}{\sigma}\sqrt{\frac{\frac{n-|m|}{2}!}{\pi\frac{n+|m|}{2}!}}L_{\frac{n-|m|}{2}}^{(|m|)}\left(\frac{r^2}{\sigma^2}\right)\times\left(\frac{r}{\sigma}\right)^{|m|}e^{-\frac{1}{2}\left(\frac{r}{\sigma}\right)^2 – im\theta}$